This is a reference to a project on CodePlex. It refers to a Silverlight 2 Beta 2 sample application (with source code) that will show solutions to a set of problems in Silverlight. E.g. creating custom controls, communicating with a WCF service, use replaceable GUI (templating), captcha functionality, creating custom panels, multi templated ListBox.
I hade the privilege to post one of my project at work on CodePlex. It is a Silverlight application where you in edit mode can create a greeting with text, images and magnetic poetry and then send it to your friends or save it to a database. The receiver can then read the message or you can embed your message on a web site. I had a lot of help from Johan Normén and Robert Folkesson in this project.
You find the project here!
Tuesday, September 23, 2008
A Greeting Creator - Silverlight
Sunday, September 21, 2008
Scale, Move and rotate controls in your GUI - WPF
In this blog post I will describe and share a custom control in WPF (Windows Presentation Foundation) that you can use to encapsulate other WPF-controls. This control will add functionality so that you can move, rotate and scale your WPF-controls with your mouse. It also adds a control that will rasie an event (Click-event) when clicked. You can download a sample project with the control at the bottom of the page.
In the image above the red square is a control that will raise a click-event. The outer gray squares are for rotation and the inner ones are for scaling. In the middle the control can be moved (translated).
Background
In a previous post I did this control in Silverlight. This is the WPF version and I have made some adjustments and used some other solutions in this version. These will be described below.
Problem
Many times when you design an application you do not only want to present information to a user, you also want the user to interact with your program and your controls. Sometimes this interaction involves moving, scaling and rotating an object. I missed a simple control to wrap other controls in that would add this.
How does it work?
Feel free to compare this solution with the Silverligh version. To start with, this is a control that can be used as a container for other controls. This means that you do not have to modify any other code in your existing controls. This control just adds the ability to interact (move, scale and rotate) with your control.
To do this we need a bock of Transforms in our custom control:
<Grid.RenderTransform>
<TransformGroup>
<ScaleTransform ScaleX="{Binding RelativeSource={RelativeSource TemplatedParent}, Path=Scale}" ScaleY="{Binding RelativeSource={RelativeSource TemplatedParent}, Path=Scale}" />
<RotateTransform Angle="{Binding RelativeSource={RelativeSource TemplatedParent}, Path=Angle}"/>
<TranslateTransform X="{Binding RelativeSource={RelativeSource TemplatedParent}, Path=X}" Y="{Binding RelativeSource={RelativeSource TemplatedParent}, Path=Y}"/>
</TransformGroup>
</Grid.RenderTransform>
This is the first different to the Silverlight version. In Silverlight we used the PART_-convension and set the ScaleX, Angle etc. in code behind through these variables. We still have dependency properties in code behind but we don't need to have the reference to the Transforms. Instead we use a binding to our properties. The RelativeSource property is something that doesn't exist in Silverlight and therefore we need the PART_-solution in that example. In WPF we can solve the problem by using a binding and in that way we doesn't restrict us as much in the template. It also results in less code in code behind (a lot of code/logic is removed) and that will make this control simpler and easier to maintain.
The templates are much the same in WPF/Silverlight. One other difference than the binding is that the VisualStateManager doen't exist in WPF (but I think it will be implmeneted in future versions). I love the VisualStateManager and its separation between control and template. In WPF we instead put all the interaction controls in a Panel and then set the Visibility of that panel in code behind.
To get all the interaction working we hook up on mouse events in both our interaction controls (panels) and also in our base the custom control (inherited from ContentControl). When I first translated from Silverlight to WPF the whole control froze after the first interaction. The listeners for mouse event looks like this:
private void OnScaleControlsMouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
_previousPosition = e.GetPosition(this);
this.CaptureMouse();
_iteractionMode = InteractionMode.Scaling;
}
We call CaptureMouse() and in Silverlight this will be automatically released then the mouse button is released. In WPF we need to do this manually by setting:
private void OnControlMouseLeftButtonUp(object sender, MouseButtonEventArgs e)
{
_iteractionMode = InteractionMode.None;
Mouse.Capture(null);
}
With Mouse.Capture(null) the mouse will be released.
WPF also have more Cursors that we can use. We use more common cursors for moving and sizing which makes the interaction more intuitive.
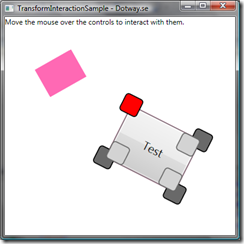
Code and sample project can be found here.
Saturday, September 20, 2008
Scale, Move and rotate controls in your GUI - Silverlight
In this blog post I will describe and share a custom control in Silverlight 2 that you can use to encapsulate other Silverlight-controls. This control will add functionality so that you can move, rotate and scale your Silverlight-controls with your mouse. It also adds a control that will rasie an event (Click-event) when clicked. You can download a sample project with the control at the bottom of the page.
In the image above the red square is the are that will raise a click-event when pressed. The outer squares at the other corners is controls for rotation and the inner squares are controls for scaling.
Problem
Many times when you design an application you do not only want to present information to a user, you also want the user to interact with your program and your controls. Sometimes this interaction involves moving, scaling and rotating an object. I missed a simple control to wrap other controls in that would add this.
How does it work?
A custom control in Silverlight and WPF is a control that contains some functionality and it is look-less. This means that you can change and switch the presentation of a control by changing its template. The base class chosen for this control is ContentControl and this base class is extremely powerful for encapsulating other controls (the content).
The functionality we want in this control is logic for clicking, moving, rotating and scaling. This includes listening to mouse-events of different kinds and the acting on those. What we need is some way of catching these events for the different type of transforms that we want to do.
<Grid x:Name="PART_TranslateControls">
<Rectangle Fill="Transparent" Cursor="Hand" />
</Grid>
<Grid x:Name="PART_ClickControls">
<Rectangle Fill="Red" Stroke="Black" Cursor="Arrow"/>
</Grid>
<Grid x:Name="PART_RotateControls">
<Rectangle Fill="DimGray" Stroke="Black"/>
<Rectangle Fill="DimGray" Stroke="Black"/>
<Rectangle Fill="DimGray" Stroke="Black"/>
</Grid>
<Grid x:Name="PART_ScaleControls">
<Rectangle Fill="LightGray" Stroke="DimGray"/>
<Rectangle Fill="LightGray" Stroke="DimGray"/>
<Rectangle Fill="LightGray" Stroke="DimGray"/>
</Grid>
The rectangles in the snippet above is only the appearance of the control and since we are listening to events from the panels we can set any look and feel of the clickable areas. Also not that we use the PART_ convention for creating custom controls.
In code behind, we add listeners for mousedown events for the different panels and calculates new position, rotation or size when the mouse is moved. The ContentControl contains a TransformGroup with a ScaleTransform, RotateTransform and a TranslateTransform which is the values that we are adjusting.
<TransformGroup>
<ScaleTransform x:Name="PART_Scale"/>
<RotateTransform x:Name="PART_Rotation" />
<TranslateTransform x:Name="PART_Translation" />
</TransformGroup>
We also have dependency properties that we can bind to in this control. The most beautiful solution for this would be to skip using PART_ for the transform and bind to the properties instead. The problem is that you can not bind to ScaleX, Angle, X etc. and therefore we need to get these parts in code-behind and then set the properties manually.
In the sample project below you can see all the mechanisms for this control.

Update 1: The sample code is now updated to Silverlight 2 RTW and I also added an sample of a transformable TextBox and functionality for ZIndex.
Update 2: The sample code has been updated. Hal9000Lives pointed out that the calculations for the rotation used the upper left corner as its center point. This has now been changed to use the middple point as center.
Code and sample project can be found here.
WPF-version of this control can be found here.
Thursday, September 4, 2008
How to use Sessions in WCF and Silverlight (basicHttpBinding)
I was writing a WCF Service for a Silverlight application and I needed to use Sessions in my WCF service to store some info. As you know Silverlight 2 (beta 2) only supports basicHttpBinding and therefore we can not use sessions as in wsHttpBinding. I wanted to write something like:
HttpContext.Current.Application.Add("MyKey", someInfo);
But HttpContext.Current returned null. After reading some threads in forums I found this workaround, make your WCF Service ASP.NET compatible:
1. Put this attribute on your WCF Sevice class: [AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Allowed)]:
[AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Allowed)]
public class MyService : IMyService
{
...
}
2. In your Web.config for your WCF Service project set:
<system.serviceModel>
<serviceHostingEnvironment aspNetCompatibilityEnabled="true"/>
</system.serviceModel>
This will make HttpContext.Current return something and you can now store your sessions. The difference I found in my solutions from some others is that I had a separate project for my WCF service than my ASP.NET web-project and that made the HttpContext fail.
Read more here: https://silverlight.net/forums/p/14175/57586.aspx